Inline Flow
- The Inline Flow makes it easy for you to facilitate Accrue payments with a streamlined UX that doesn't take users away from checkout.
- Users will be able to create a payment intent and enter an additional payment method (if paying by wallet).
- Accrue will handle all the balance checks and funds aggregation, allowing you to confirm a Payment Intent within your app.
Client ID
Your dedicated account manager at Accrue will provide you with Client IDs for all your integrations. The Client ID
will look similar to this example: 123e4567-e89b-12d3-a456-426614174000
Pay by Wallet
To provide a better experience for users, the inline flow for PBW (Pay by Wallet) can accept the wallet widget data to be passed to the Accrue Pay main script.
Refer to the Loading widget data guide for more details.
Accrue JavaScript Loaded (Controlled)
- This is our recommended integration method, since we will be able to optimize the user experience for you, with minimal work on your side.
- You can use
<accrue-pay flow="[bank|wallet]"></accrue-pay>
to indicate the placement. - Please refer to Client Configuration on how to define additional custom placements.
<html>
<head>
<script
data-script="accrue-pay"
defer
src="https://pay[-sandbox].accruesavings.com/main.js"
data-client-id="{your-client-id}"
data-wallet-widget={widget.attributes.payload}
></script>
<script>
document.addEventListener("AccruePay::Ready", () => {
window.accruePay.onGetUserData(() => {
// Your own user data to be attached to the user profile stored with Accrue
return {
referenceId: "123e4567-e89b-12d3-a456-426614174000",
email: "user@email.com",
phoneNumber: "+1212559999",
};
});
window.accruePay.onSignIn((payload) => {
// Once the user signs in you'll receive basic profile data that you can store and use for lookups
// return {
// id: "497f6eca-6276-4993-bfeb-53cbbbba6f08",
// referenceId: "123e4567-e89b-12d3-a456-426614174000",
// isNewUser: true,
// };
});
window.accruePay.onPaymentIntentStart(() => {
// Send the current cart total amount to Accrue Pay.
return {
walletId: "123e4567-e89b-12d3-a456-426614174000", // Only required when implementing `Pay by Wallet`
amount: 9999, // Positive integer representing how much to charge in the smallest currency unit (e.g., 100 cents to charge $1.00)
reference:
"Cart ID, User ID, or any freeform combination of IDs or random strings",
};
});
window.accruePay.onPaymentIntentUpdate((paymentIntent) => {
// Store the created payment intent into a form field or local checkout state.
console.log(paymentIntent);
});
});
function onPay() {
// Make a request to your backend
// Include the `paymentIntent.id` in the request body
// As part of your backend logic use the `paymentIntent` to make a request to the `Accrue Merchant API,` then authorize and capture the payment
// Please refer to the `Merchant API Integration` section for more details
}
</script>
</head>
<body>
<accrue-pay flow="[bank|wallet]"></accrue-pay>
<button onclick="onPay()">Pay</button>
</body>
</html>
Updating the amount dynamically
Whenever you need to update the amount after the initial load of the SDK, simply call the setAmount
method:
window.accruePay.setAmount(9999); // Positive integer representing how much to charge in the smallest currency unit (e.g., 100 cents to charge $1.00)
Updating widget data dynamically
Whenever you need to update the widget data after the initial load of the SDK, simply call the setWalletWidgetData
method:
window.accruePay.setWalletWidgetData("{payload}");
Updating the subtotal
Should your rewards be based on a subtotal (like amount before taxes and/or shipping) rather than the cart total, you can use the setSubtotal
method. This will update the reward amount shown to the user:
window.accruePay.setSubtotal(9999); // Positive integer representing how much to charge in the smallest currency unit (e.g., 100 cents to charge $1.00)
window.accruePay.setSubtotal(undefined); // To clear the value and use `amount` to calculate rewards
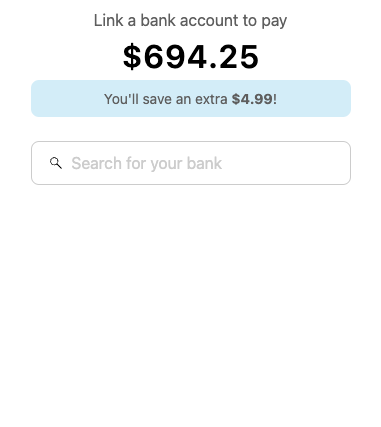
Total: $694.25
Subtotal: $499.00
Rewards: 1%
No Accrue JavaScript Loaded (Uncontrolled)
- If, for any reason, you would rather not load any of our JavaScript code to your checkout page then you can construct the iFrame yourself.
- Please make sure that iframe is rendered properly on your page, using the proper source URL layout:
https://pay[-sandbox].accruesavings.com/_/[flow]/?client={your-client-id}&amount={cart-total-amount}&reference={freeform-reference}
- Please make sure you are using the appropriate
flow
:bank
for Pay by Bankwallet
for Pay by Wallet
- Please make sure that you are listening to the
AccruePay::PaymentIntentUpdated
event. - When flow is
wallet
, you'll need to send the wallet widget data to the iframe. Refer to the Loading widget data guide for more details.
<html>
<head>
<script>
window.addEventListener("message", (event) => {
if (
event.origin === "https://pay[-sandbox].accruesavings.com" &&
event.data?.key === "AccruePay::PaymentIntentUpdated"
) {
const paymentIntent = event.data.paymentIntent;
// Store the created payment intent into a form field or local checkout state.
console.log(paymentIntent);
}
});
function onPay() {
// Make a request to your backend
// Include the `paymentIntent.id` in the request body
// As part of your backend logic use the `paymentIntent` to make a request to the `Accrue Merchant API,` then authorize and capture the payment
// Please refer to the `Merchant API Integration` section for more details
}
function loadWalletWidgetData() {
// When flow is `wallet`
// Send the wallet widget data to the iframe
// Refer to the Loading widget data guide for more details
try {
const iframe = document.querySelector("#accrue-pay");
iframe.contentWindow.postMessage(
{
key: "AccruePay::WalletWidgetDataUpdated",
data: { payload: "{payload}" },
},
"https://pay[-sandbox].accruesavings.com" // Target iframe's origin
);
} catch (err) {
console.error(err);
}
}
</script>
</head>
<body>
<iframe
id="accrue-pay"
title="Accrue Pay"
width="340px"
height="420px"
style="border:none;"
onload="loadWalletWidgetData()"
src="https://pay[-sandbox].accruesavings.com/_/[flow]/?client={your-client-id}?wallet={wallet-id-when-pay-by-wallet}&amount=9999&reference=optional-reference"
></iframe>
<button onclick="onPay()">Pay</button>
</body>
</html>